We can always write tests against our Domain Layer or the Persistance Layer using NUnit. But what about ASP.NET pages. Luckily, we have NUnitASP which is used to write tests against ASP.NET pages (typically code behind).
We can always write tests against our Domain Layer or the Persistance Layer using NUnit. But what about ASP.NET pages. Luckily, we have NUnitASP which is used to write tests against ASP.NET pages (typically code behind).
You can download NUnitASP using the following link:
http://nunitasp.sourceforge.net/
Include the necessary libraries and you are ready to test your ASP.NET pages. Check out a simple page below which I need to test.
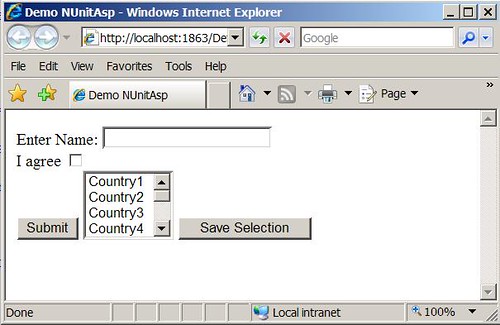
There are two parts of the page. In first part the user must fill the textbox and check mark "I agree" and press the submit button. Second part deals with selecting multiple items from the ListBox and then pressing the "Save Selection" button (I will discuss the second part in my upcoming article).
My first part looks something like this:
[TestFixture]
public class DefaultPageTestFixture : WebFormTestCase
{
public const string DEFAULT_PAGE = "http://localhost:1863/Default.aspx";
[Test]
public void CanAcceptAgreement()
{
TextBoxTester textbox = new TextBoxTester("txtUserName");
CheckBoxTester checkbox = new CheckBoxTester("chkAgreement");
ButtonTester button = new ButtonTester("Btn_Agreement");
Browser.GetPage(DEFAULT_PAGE);
Assert.AreEqual(String.Empty, textbox.Text);
Assert.IsTrue(!checkbox.Checked);
textbox.Text = "AzamSharp";
checkbox.Checked = true;
// click the button
button.Click();
Assert.IsNotEmpty(textbox.Text);
Assert.IsTrue(checkbox.Checked);
}
}
my class inherits from the "WebFormTestCase" which gives me easy access to the HttpContext object. In the CanAcceptAgreement method I created the "TextBox", "CheckBox" and the "Button" controls. Browser.GetPage([URL]) will make a request to the page.
When the page is loaded I first check that the textbox is empty and the checkbox is not checked. After that I enter the text in the textbox and check the checkbox. The button.Click will fire the button click and after that I check if there is text inside the textbox and if the checkbox is checked.
NUnitASP gives up the flexibility to check our pages without manually going through the page and checking the controls.