Widgets have gained popularity over the years. There are many websites that allows the user to add widgets on their home page. In this article we will create a simple Stock widget for our ASP.NET application using the RenderAction method.
What is RenderAction?
RenderAction allows the page to call an action of the controller. The result of the action is injected into the page. It is a good practice to treat the injected view from RenderAction as independent sections on the page.
Stocks Service:
In real world application you should use a stock service which will provide you with updated stock quotes. For this simple demo we will use an XML file to provide the changing stock information. Take a look at the structure of our XML file below:
There are three stocks in the StockUpdates.xml file. Each stock has a Name, Price and LastPrice. In order to make the application visually appealing we will also change the color of the stocks depending on their market fluctuations. Let's start by creating the model for our application.
Creating the Stock Entity and Stock Service:
The stock entity will be populated from our XML file. The entity consists of the three fields as shown below:
The Stock Service is responsible for reading the stock information from the "StockUpdates.xml". The implementation is shown below:
The GetLatest method is used to fetch all the stocks from the XML file and populate the List<Stock> collection. Now, we need to implement the view which will display the stock information.
Implementing LatestStocks Partial View:
Since, the stock information will be displayed as a small section on the page it is a good idea to render it as a partial view. If you are not familiar with partial views then check out the following article and a screencast to get started.
Understanding Partial Views in ASP.NET MVC Application
Screencast: Introduction to Partial Views in ASP.NET MVC Application
The implementation of the LatestStocks partial view is shown below:
There are couple of interesting things to note about the LatestStocks partial view. First it is a strongly typed view which is represented by the following line of code:
Another important thing to note is that everything in the partial view is contained inside the "DIV" element "divLatestStocks". The ID of the DIV is used to populate the updated stock information as we will see later in the article.
Implementing Controller Action:
The controller action is called "LatestStockUpdates" which is responsible for populating the stock list. The stock list is passed to the partial view using the ViewData dictionary. The implementation is shown below:
The final part is to invoke the Html.RenderAction method to render the controller's action on the page.
Invoking Html.RenderAction Method:
The RenderAction method can be called any where on the ViewPage. The resultant view is injected into the original page. The call to RenderAction is shown below:
If you run the page you will get the following output:
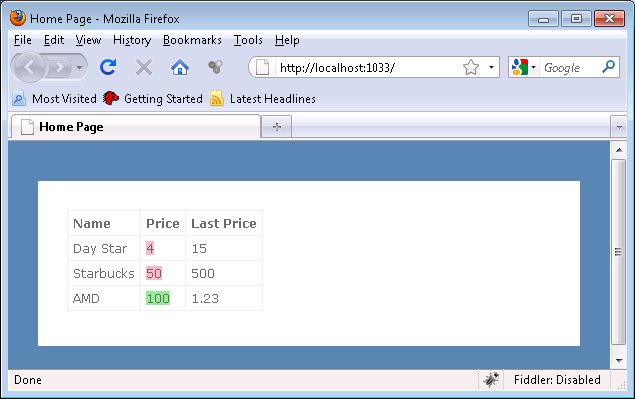
The red and green color indicates the market fluctuations. If the Price is greater than the LastPrice then it is indicated by green color. If the Price is lower than the LastPrice then it is indicated by the red color.
Currently our display is static which means even if the XML file is changed you will not see any updates on the user interface until you load the page again. We can use a polling technique to update the user interface with the latest information from the XML file.
Updating Stock Widget Using Ajax:
We will use the JQuery Ajax API to create ajax requests but you are free to use any framework you desire. The code below shows how to invoke the LatestStockUpdates controller at regular intervals.
By now you might have seen the use of the "divLatestStocks" DIV element. The main purpose of the container DIV is to update the element with the updated HTML. Take a look at the animation below:
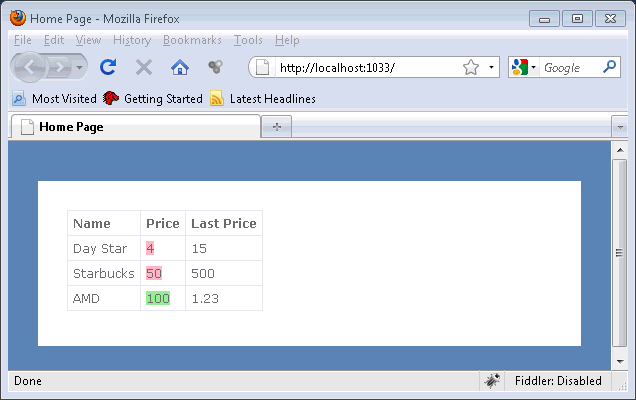
Conclusion:
In this article we demonstrated how to create a simple stock widget control using ASP.NET MVC framework. We used the RenderAction method which injected the view into the page. In the next article we will demonstrate how to create reusable components in ASP.NET MVC application which can be shared across projects.
UPDATE:
A reader suggested that instead of inserting the stocks collection into the ViewData dictionary we can simply pass the information using the second parameter to the View method. The code below reflects the change. We have also added a check for stock items. If the items are not found then an empty view is returned.
[Download Sample]