In both the previous articles we manually created documents and inserted into the MongoDb database. Unfortunately, this technique will not work in real world since we are surrounded by objects. This article demonstrates how to implement a simple business object to Document converter.
Why Creating a .NET Object to Document Converter?
Implementing a business object to a Document is not a necessity but it will tremendesly benefit the project in the long run. When working using the .NET framework everything is an object. It would be nice if we can store our .NET objects as documents in the MongoDb database.
You might be tempted to store your objects using the following code:
There are couple of problems in the above code. First, you can only refer to a single object with "Category" key. If you insert another object with the same key then the previous object will be replaced by the new one.
Another disadvantage of storing the object as a value to a document is that the MongoDb would not be able to index it properly. MongoDb prefers that you flatten out your objects and store them in the dictionary format so that each key correspond to a single value.
Implementing ToDocument Extension Method:
Instead of creating a helper or a different class to handle the conversions we created an extension method to convert our business objects into a Document. The implementation is shown below:
The above code perform recursion and populates the Document object correctly with the values from the business object. It also takes care of nested objects. Now, your conversion is as simple as the following code:
The ToDocument extension method will convert the "category" object into the following structure:
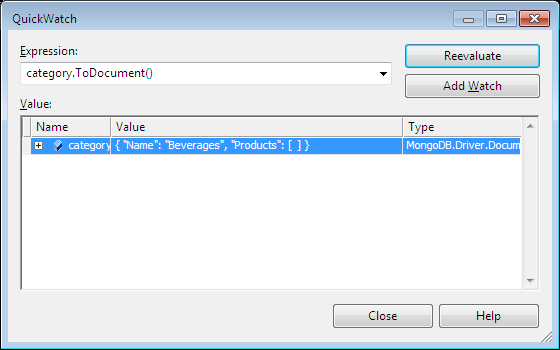
You can even convert objects that consists of nested objects. Take a look at the code below where we converted a category object which contains a products collection.
The string above represents the structure of the category object after it has been converted into the document. The format above is JSON and it is stored as BSON in the MongoDb database.
Performing the conversion between an entity and document is only one half of the story. In the next section we will discuss how to convert back from document to our entity class.
Converting Document back to Entity:
The document represents the object as a JSON string. This makes it easy to get the object back since we only have to deserialize the JSON string back to the object. We have created a ToClass<T> extension method which allows us to convert a document back to an entity class.
You can use any JavaScript serializer to deserialize the JSON string. We are using the JavaScriptSerializer contained in the System.Web.Extensions assembly.
One very important thing to note when deserializing objects is to have all the properties which you want to deserialize have a setter. That is why our Category class has Products property with a setter as shown below:
Here is an example of using the ToClass<T> method.
The above code will fetch the document with the value "Liqior" as the "Name" property and then convert it into the category object.
References:
1) MongoSerializer
Conclusion:
In this article we demonstrated how to create a simple Object to Document converter. This greatly minimized our conversion code and now we can focus on working with our business objects and storing them as documents in the MongoDb database.
[Download Sample]