In one of the previous articles we implemented a Twitter search application. The application utilized the AFNetworking library for request handling and SDImage for handling image requests. In this article we are going to go back to the basics and use the classes available in the iOS 5 framework to build the same application.
Implementing TwitterService Class:
The first task is to implement the TwitterService class which will make the actual request to fetch the tweets. The TwitterService takes in the URL parameter which contains the searched keyword. The TwitterService implementation is shown below:
TwitterService.h:
TwitterService.m:
The TwitterService class can be utilized from the view controller as shown in the following implementation:
Unfortunately, there is no way for the view controller to know that the request has been completed successfully. There are multiple ways of solving this issue but we will be using the power of delegates to communicate from the TwitterService to the view controller.
The TwitterServiceDelegate implementation is shown below:
The updated connectionDidFinishLoading is shown below:
Inside connectionDidFinishLoading we invoke the didReceiveTweets delegate method which is implemented in the TweetsViewController controller as shown below:
In the TwitterService class we used the TweetHelper class to convert the JSON data into a Tweet model class. The TweetHelper implementation is shown below:
NSJSONSerialization is a new class introduced in iOS 5 which enables easy parsing of JSON data. The Tweet class uses the custom initWithDictionary method to convert the dictionary into a Tweet model class.
The Tweet class is implemented below:
The setValuesForKeysWithDictionary method automatically matches the dictionary values to the object’s properties. The setValuesForKeysWithDictionary matches the dictionary keys with the property names. If the key is not found then the forUndefinedKey method is triggered where you perform custom mapping between the key and the property name. The setValuesForKeysWithDictionary method should be utilized when there is not a big difference between the dictionary keys and the model properties, otherwise you will be performing a lot of custom mapping inside the forUndefinedKey method.
Implementing TweetCell:
If you are going to use a custom UITableCell with UIImageView then you will realize that when images are being fetched the complete user interface freezes. This is because the images are fetched on the same thread. In order to make the image fetch operation in the background we can utilize the power of GCD (Grand Central Dispatch).
We are going to extend the UIImageView class by using Categories which will support the fetching of image on a separate thread. The Category is implemented below:
We used the dispatch_async block to fetch the image and then use a second dispatch_async to update the main thread which in turn updates the user interface. The result is shown in the screenshot below:
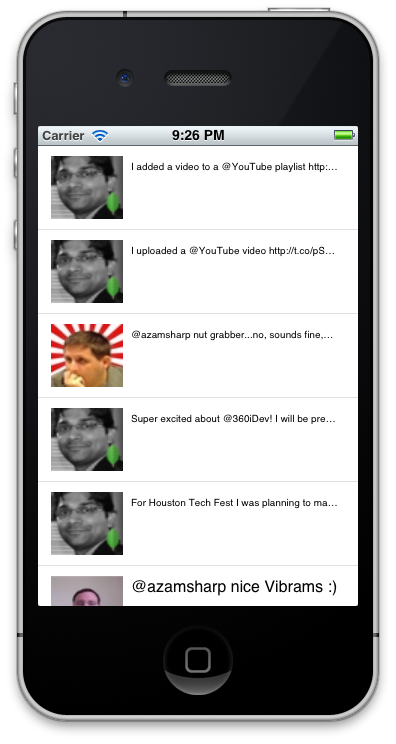
Recommended Reading:
1) Implementing Twitter Search iOS App Using AFNetworking, UITableView and MBProgressHUD
Conclusion:
In this article we learned how to utilize many interesting features of the iOS SDK. These features include GCD, Categories, NSJSONSerialization etc.